Description
This is both a game engine and a game editor I've written in C# with XNA and WPF. The engine is component based like Unity Engine. The editor uses MVVM design approach (Model View ViewModel).
MVVM means decoupling the UI and the logic to maintain the code easily and do unit testing, also allows the designer to work independently from the developer.
______________________________________________________________________________________________________________________________
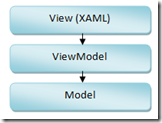 |
View in this case is the XAML code that builds the UI, basically what you see when the software runs.
ViewModel is all the C# code that runs the functionality and stores and processes all the data. It's basically a mirror of the View (the UI) that can run standalone
Model is the actual data that the ViewModel interprets and shows in the View such as a material or a texture. |
______________________________________________________________________________________________________________________________
The communication between the View and the ViewModel is done through the feature called "data binding" that ships with WPF, but also with alot of user developed extensions to make MVVM easier.
The final "game" is built using the MSBuild class to generate a visual C# project in memory and compile:
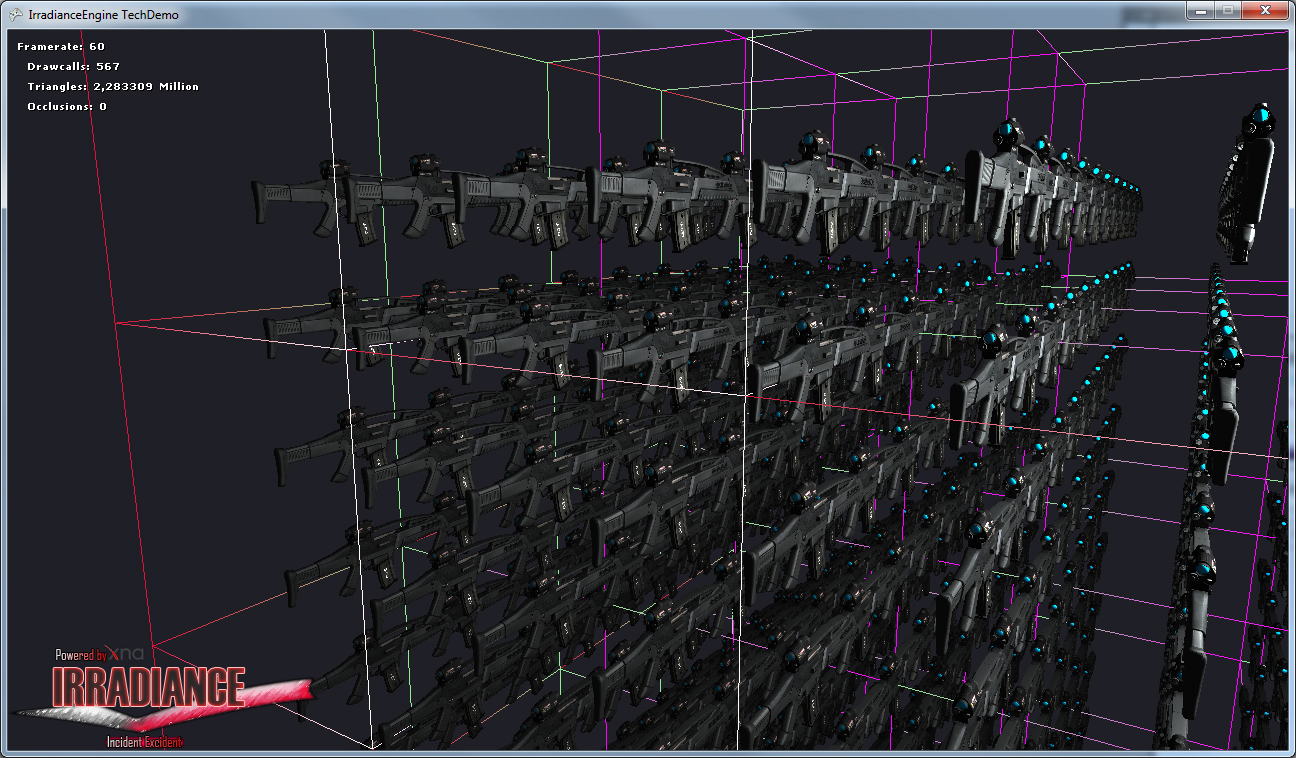
There is so much I could write about this tool. This is propably the project that I have learned the most knowledge in a short amount of time compared to all my other projects.
Coded my own propertygrid, assetmanager etc. The part that I'm most proud of is the shader importer. It scans the .fx file and creates a C# class that has getter and setter methods for all the shader constants. It also checks for engine used semantics for lights, matrices and fills the method SetEngineParameters(...) and SetLightParameters(...) that are inherited from the IShader interface. Once the C# file is generated it compiles it at runtime so it can be used in the engine :)
When building out a game, it already has the C# file so it just includes it in the memory generated Visual C# project and compiles it :)
Rendering pipeline
Note: OPTIONAL means can be set on or off!
Update active objects
Camera frustum culls octree nodes
Camera frustum culls objects that are left but outside screen (OPTIONAL)
Engine fills ZBuffer early on with Front to Back sorting (OPTIONAL)
Drawing with 3 possible settings (OPTIONAL Occlusion Query culling)
1. Sorts and batches material / renderstates wise and loops inside the effect pass and does the drawcalls
2. OR Uses the order left by the ZBuffer sorting
3. OR Uses the order from the scenegraph
Handles Transparent objects back to front
Further development
I've been working on integrating a light prepass renderer as a deferred rendering solution.
I'm also working on integrating Hardware instancing with Shader Model 3 (extra vertex buffer holding the transformation matrices)
I'm working on this whenever I have time, engine development never stops =}